Your First API Call
The first step in making an API call to the EPC platform is getting authorized for access by obtaining a Partner OAuth Token.
OAuth 2.0
The Encompass Partner Connect Platform (EPC) uses OAuth 2.0 for authentication and authorization. OAuth enables a client application to gain delegated access to information on behalf of the user without disclosing their credentials. If you are new to OAuth, you can learn more from the OAuth 2.0 specification guide, or one of the many beginnerβs guides available online.
Creating a New Authentication Token
To obtain an OAuth token, use the Postman collection you downloaded and configured in your Postman client to make a POST
operation to the https://api.elliemae.com/oauth2/v1/token
endpoint. This request can be found in the sample EPC Postman collection. Find the Create Partner Authentication Token request in the Authentication and Authorization folder, as shown below.
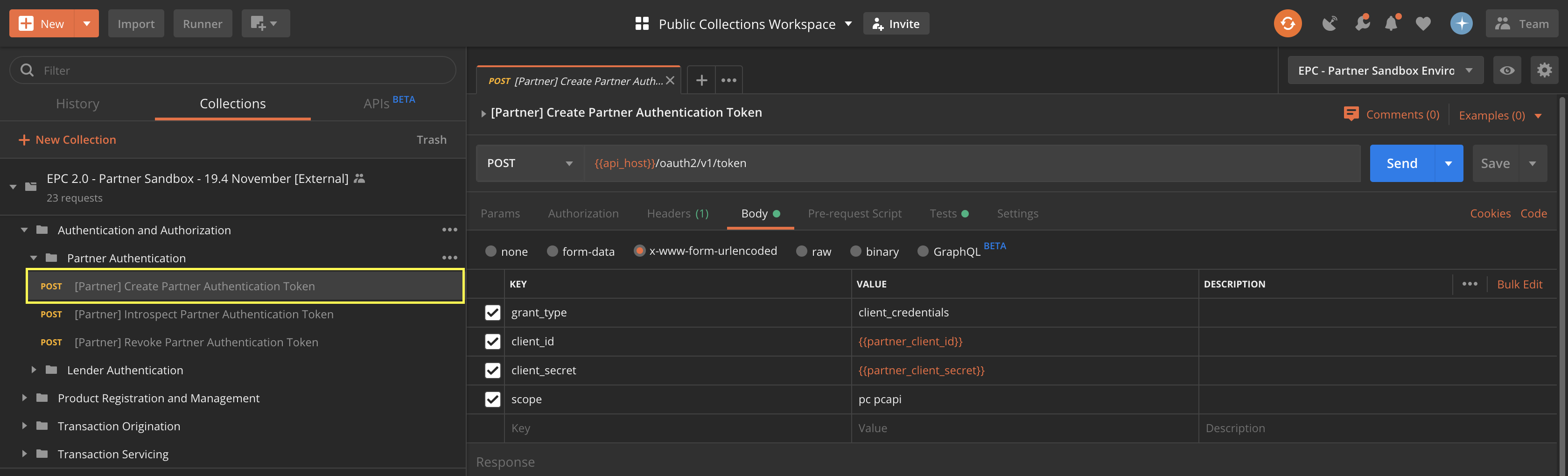
POST /oauth2/v1/token HTTP/1.1
Host: api.elliemae.com
Content-Type: application/x-www-form-urlencoded
grant_type=client_credentials&client_id=<=CLIENT_ID>&client_secret=<CLIENT_SECRET>&scope=pc+pcapi
If your environment variables are configured correctly, you will not need to manually input parameters. Just click the Send button. A successful response will look like this:
{
"access_token": "PDVF2zWjJcR1s0WwHabYxDod3BB4",
"token_type": "Bearer",
"expires_in": 7200
}
The attribute access_token
holds the value of the OAuth token. The request in the EPC Postman collection is set up to copy the value of access_token
to the partner_token
environment variable. After you get a valid token, you can immediately make other EPC calls. You do not have to manually supply the value of access_token
to other requests in the Postman collection.
oAuth Access Token Life-time
This token will expire in two hours. The value of
expires_in
, 7200, is in seconds. Making calls using this token will not extend its life.
The following Python code snippet illustrates how to receive the same response in your EPC application:
import requests
url = "https://int.api.ellielabs.com/oauth2/v1/token"
payload = f"grant_type=client_credentials&client_id={CLIENT_ID}&client_secret={CLIENT_SECRET}&scope=pc%20pcapi"
headers = {
'Content-Type': "application/x-www-form-urlencoded"
}
response = requests.request("POST", url, data=payload, headers=headers)
print(response.text)
Introspecting an Existing Authentication Token
You can introspect the authentication token you created by making a POST
request to the https://api.elliema.com/oauth2/v1/token/introspection
endpoint. This request is under the Authentication and Authorization folder in the sample EPC Postman collection, as shown below:
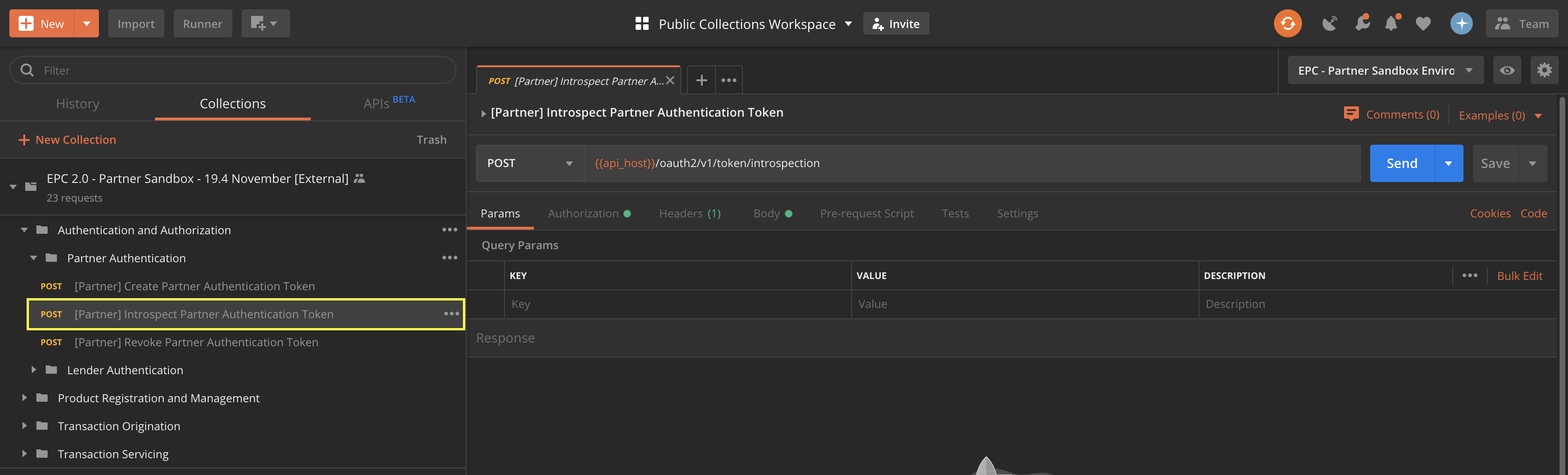
Content-Type: application/x-www-form-urlencoded
Authorization: Basic YTVkcXJoYzprRnluSSFoQERhMTNLdF5VSDNtS2dEZ0M5MHloYWZnaSFkaXk1TGs5emJyejhWTmskRGNmM1RLTXN1YzVxTVcj
token=g3BZnPN7ONIC6YXP3NnW0zUHGY5d
You will see the following response body to your request:
{
"active": true,
"scope": "pc pcapi",
"client_id": "{{API_CLIENT_ID}}",
"token_type": "Bearer",
"exp": 1577155896,
"environment": "Test",
"encompass_instance_id": "Test",
"identity_type": "Partner",
"encompass_client_id": "{{PARTNER_ID}}"
}
Partner ID
Keep a copy of the returned
encompass_client_id
attribute on hand. This is your unique Partner ID with Ellie Mae. You will need it in subsequent API calls made in this exercise.
Updated about 4 years ago